Today I am going to show you a software that I just made with Laravel. It is a sales system, point of sale, POS or whatever you call it, which is used to keep track of the products that are sold, the sales, and so on.
It is a totally free and open source system; which means that you can use it at no cost, and modify it to your needs or customize it. Among its features we find:
- It Uses Laravel in its latest version (as of this writing)
- Bootstrap is used for styles
- FontAwesome Icons
- Fully responsive system, that is, it works on mobiles, tablets and computers
- Inventory control with sale price, purchase price, profit, stock, etc.
- Option to make sale, adding products
- Checking inventory stock when selling
- Subtraction of stock when selling
- Printing of sales tickets on thermal printer
- Sales report
- User login and registration
- User management
- Customer registration
- Ticket that includes the customer’s name
Now let’s see how I have developed it, where you can download it, and so on.
Sales system design
Laravel uses Blade for the templates, so I have used their system of layouts and inheritance. I define the master template which is based on a Bootstrap 4 starter template:
So now I just have to overwrite the content. From the template you can see that I show a navigation menu and that I hide some elements in case the user is not logged in.
Routes
We have several routes configured to show interfaces for selling, view sales, print tickets, view products, and so on. All of them are protected with the auth middleware, so they are not allowed to be accessed if you have not logged in.
The web.php file looks like this:
In it, the controllers that are resources are registered, as well as other routes such as login, registration, add product to the shopping cart, and so on.
Login to the system
I have used Laravel’s default authentication, so to use the sales system written in PHP & Laravel you have to log in:
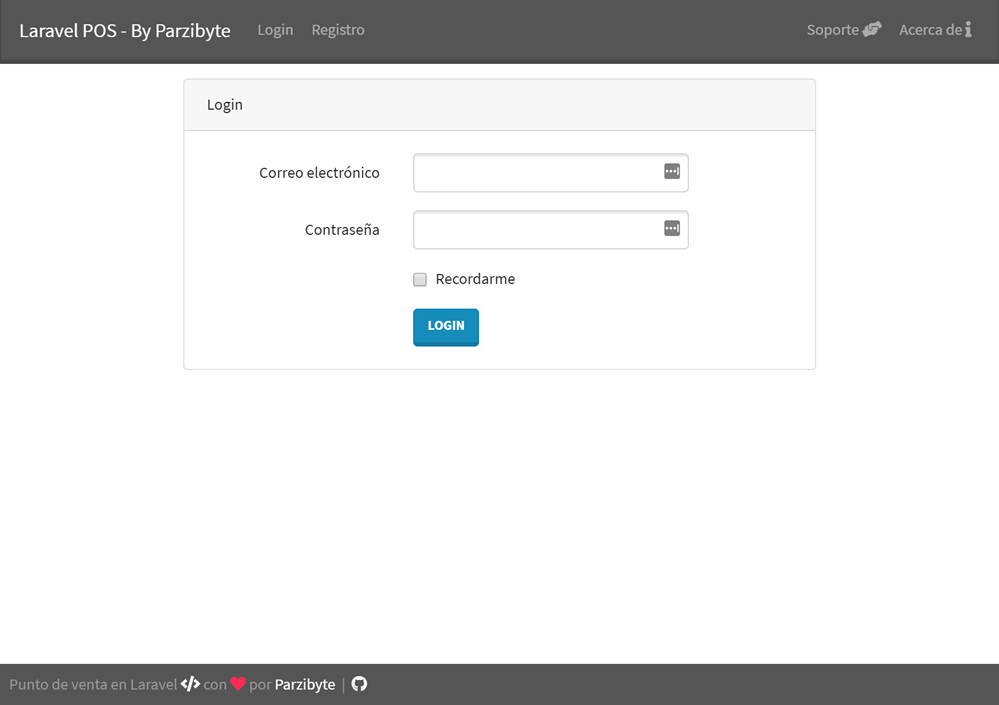
In the same way, a screen is provided to register (so you can register your user and then access the POS), which can be disabled as desired.
Welcome screen
When you log in you are redirected to the index or welcome screen. It shows shortcuts to the sections of the system:

The index screen code is as follows:
I am using Bootstrap cards. And from that you can navigate to the following sections.
Product management – Inventory
We have product management with Laravel where the stock, the purchase price, the sale price, the barcode and the item description are recorded. The definition of the model and its fillables looks like this:
The form to create a new product is as follows:
It is a simple form that will lead to the following controller or product controller, which in turn is a resource:
The edit form is very similar to the insert form. To display the products, a responsive Bootstrap table is used in addition to using Blade’s @foreach
:
With the result shown in the picture below:

Remember that the full source code can be seen on GitHub; in the end I will leave the link. From here on I will not fully explain the repetitive code, since I already did it with the products. Let’s look at the things that are most important.
Making sales
Interface to sell
In the interface we have a field that is used to scan the barcode, whether a reader is used or the code is written and the Enter key is pressed.
When there are products, the list of them is shown as well as some buttons to cancel the sale, end the sale or remove a product from the sale list.

In addition to that, the total of the sale formatted as currency is displayed.
List of products sold
The shopping cart or list of products for sale is saved in the session as an array, in this way it is not lost even if the user refreshes the page or navigates to another place; thus allowing better control.
I have implemented a simple shopping cart with an array, the functions that handle it are:
It handles various scenarios. For example, when a product is added, the barcode is sent and a product is searched by that code; then two verifications are made:
- That there is existence of the product
- That when adding the product to the sales list it will not run out of existence
For example, if you want to sell more than what exists, a warning is displayed:
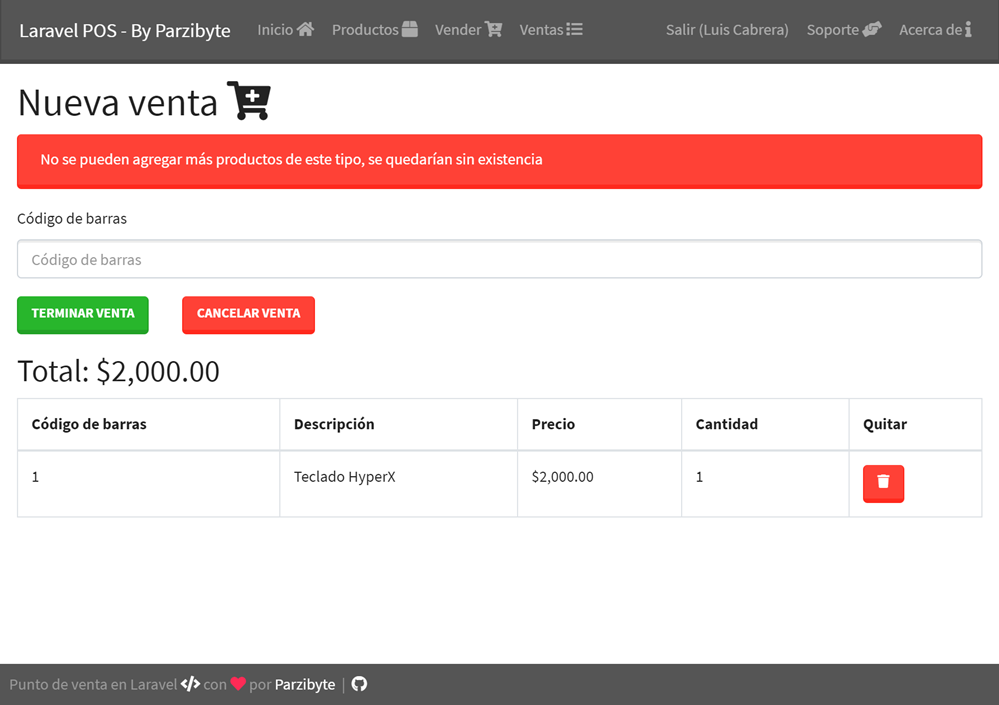
Finish sale
At the end of the sale several things are involved. Starting with that we have a different model called ProductSold (ProductoVendido) that is responsible for encapsulating how a sold product behaves.
I have separated it this way because the sale price will not always be the same; that is, today you can sell a product for 100 dollars that tomorrow will cost 112 for example; then the selling price at which it was sold must be saved for the record.
In addition to that, the product has a relationship with the Sales (Ventas) table, which have a creation date (the timestamps) and an id:
Here’s an interesting point, as we have a relationship between products and sales, using the relationships that Laravel provides. Finally, to finish the sale, the following is done:
And this is how sold products are saved along with the sale and creation date. Later we will use this to view the sales report, the details of a sale and to print tickets.
Sales report
The sales report shows the total of the sale, the date of sale and buttons to view the details, print the ticket or delete the sale. The query to list the sales with the total is:

When the sale detail is shown, we use the controller’s show
method, which shows a sale. There, thanks to the relationship that we defined previously, we can obtain the product table and draw the detail as the total:

By the way, the view is as follows:
That is what is responsible for drawing the table, placing the links, and so on.
Sale ticket
Note: if you want to know how to configure your printer see the post on how to print a ticket in PHP.
Let’s see how the ticket is printed on a thermal printer. What you have to do is install the mike42 library; I was based on my tutorial to print on thermal printer with Laravel.
The controller’s function is as follows:
We start by obtaining the sale by ID, the same variable that is passed through the URL. The name of the printer is in the .env
file, so it can be easily configured.
We print the header, the date of the sale and then we go through the products of the sale (previously registered in the database). For each one we draw the quantity, the description and the subtotal.
At the bottom of the ticket we print the total and a thank you message. Later it is redirected to the page it came from and if everything is fine, the ticket should look similar to the one in the following image:
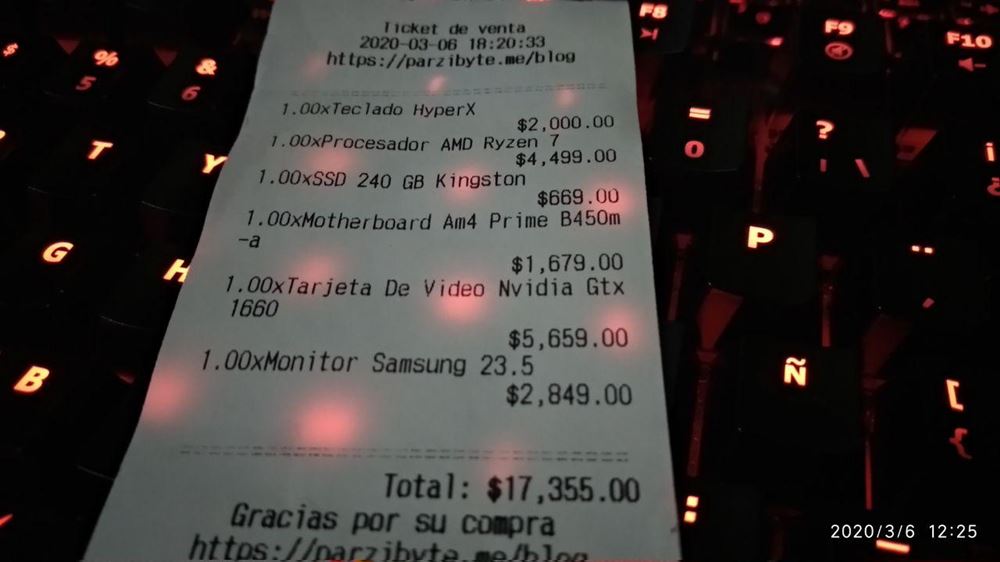
Obviously the ticket will change depending on the list of products, and it is totally dynamic.
Responsive sales system
I want to show that this system adapts to any screen size. For example, on a mobile phone it looks like this:
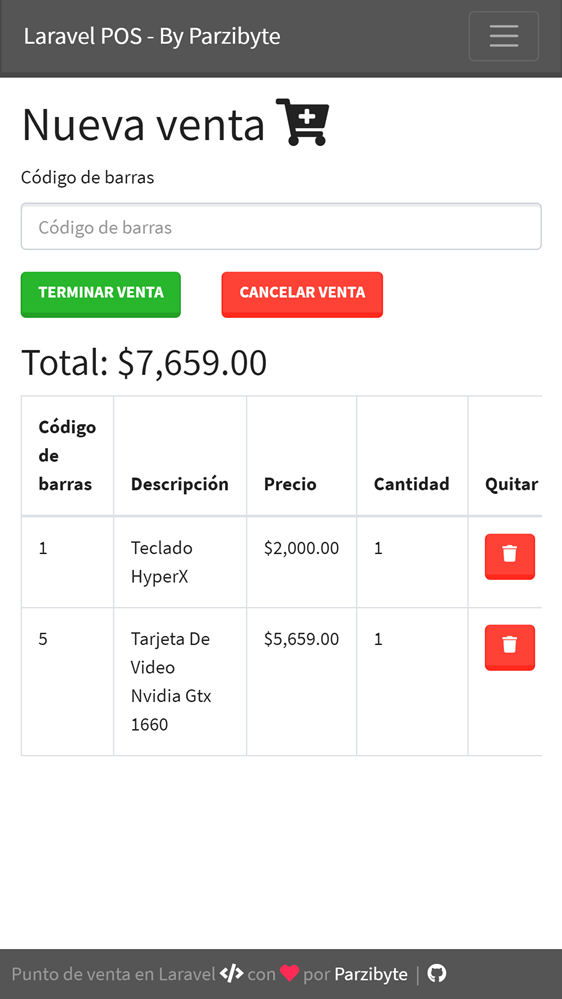
Therefore you can use it in a local area network or upload it to a hosting; you can visit it from anywhere.
Download software and view source code
The source code for the program is in my GitHub repository. If you want to download the code, click on Clone or download> Download ZIP within the repository. To use it, configure the env file, install the dependencies with composer and make the necessary migrations with php artisan migrate
.
Installing system
You can watch this YouTube video where I show you how to install this free laravel based system:
Demo
You can watch a video where the system is in action:
You can also test it online.
Conclusion
For a class that I am currently taking at the university I had to do a CRUD with Laravel, but I had wanted (for a long time) to make a point of sale with Laravel and add some things like ticket printing.
If you like the framework, I invite you to read more about Laravel on my blog. I also invite you to see my other projects, some of them open source.
Note: I have added more modules to this system, including the clients module and the users module.