In this post I will show you how to program the Connect 4 game by using JavaScript with HTML and Vue, with Bootstrap styles.
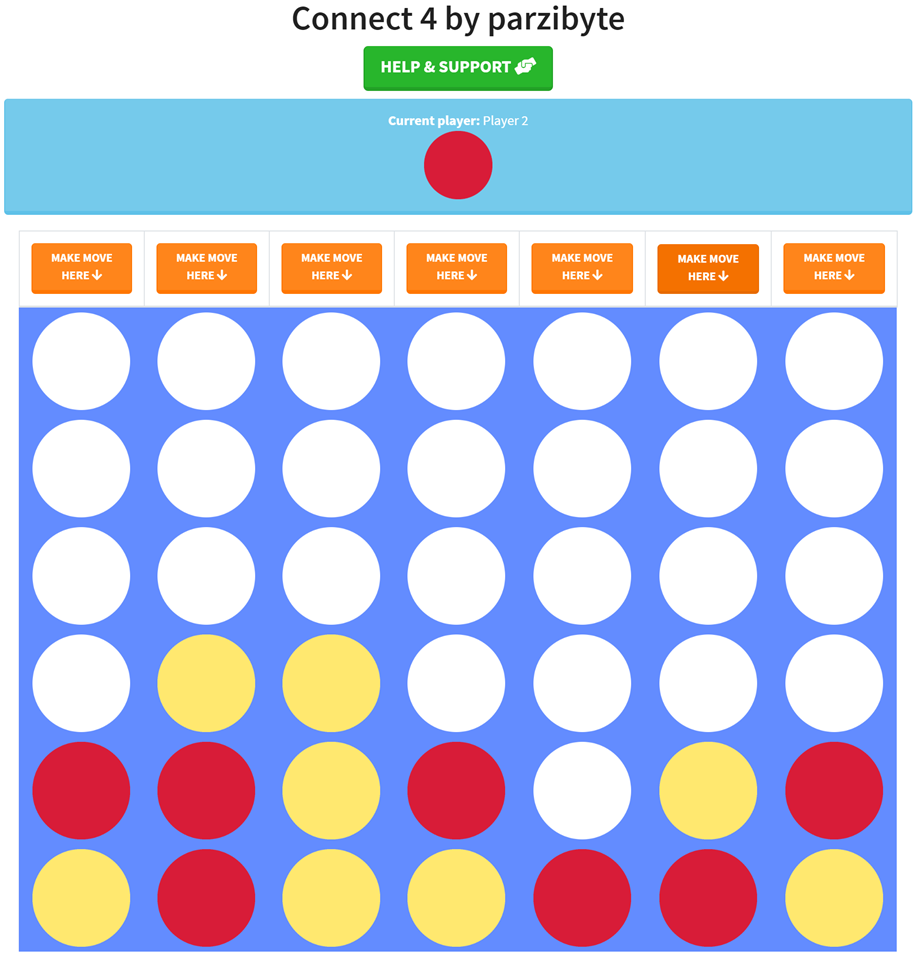
It is the Connect 4 game but web version with a player versus player option, as well as player versus CPU that uses a small artificial intelligence.
Throughout the post I will show you how the game works, what technologies I have used, styles, etc. I will also show you how to download the source code, as the game is totally free and open source. Finally I will leave you a demo to play connect 4 online.
Game board
The game board is a dynamic table that is drawn from an array. I have removed the borders and reduced the padding, as well as adding a blue background color to to make it look like the real game board (i.e. the real world, the physical one).
Inside each cell of the table (or td) I have placed an image that can be:
- Empty space
- Player 1
- Player 2, who is the CPU when playing against him
These images can be changed either in code or actually on the file system. The image path is returned by a function that, depending on the value, returns a different image.
Drawing game board
As I said earlier, the board is a table that looks like this:
The table header has a button that causes the piece to be placed in that column, that is, it drops the piece.
Because it is a two-dimensional array or a matrix, we first go through the entire array to get the row. And within that row we repeat a cell for each value in the row.
In each cell there will be an image that will have as source what the cellImage
method returns, which we will see next when we see the programming in Vue.
That’s all in terms of interface design, it’s time to see the JavaScript code for this Connect 4 web version game.
Image
The method that evaluates the image and returns the path is the following:
Like I said, it is a simple if
. I left it that way to be expressive, although if you want, you can use dictionaries, concatenate the cell, etc., as well as use different images.
Customizable constants
We can define the size of the board or the number of pieces that must be connected, in this way we could play Connect 3, Connect 5, and so on!.
If you notice, when you play connect 4 against the CPU, it is taken as player 2.
Reset game
We have the following function that resets the game. What it does first is ask the game mode: player versus player, or player versus CPU.
The next step is to clean the game board, filling it with empty spaces. Then, select a random player, who is the one who takes the first turn.
Finally it tells the CPU to make its move in case it is its turn.
Make user move
When the player or user presses the button to place the piece, the following code is executed where it is validated if the column is not yet full, and it is checked if there is a tie or if the player has won.
The method receives the column number.
The piece is placed in the matrix, using the indicated column (minus 1) and in the empty upper row. In other words, the value in that cell is simply changed and Vue automatically takes care of refreshing it in the table.
Finally, the player is exchanged and the CPU is instructed to make its move in case it is its turn and the game mode is CPU versus player.
By the way, in case there is a draw or a winner, the user is asked if they want to play again. In that case, the game is restarted.
Know if there is a winner
In this game there is a winner when there are 4 pieces connected in any direction in a straight line. Therefore, you simply have to count in all possible directions to see if there is a Connect 4 in the matrix for the player in question.
For this I have created some functions that perform the counting in several directions:
What the code does is to walk the board from one point to another and increase a counter in case it finds adjacent pieces. If it finds a piece that is not the same color, then it resets the counter to zero.
The function that decides if there’s a winner is the following:
What it does is count in all directions, and if it detects that there is a row followed by connecting pieces that are greater than the number needed to win, then it returns true
. Otherwise false
.
Tie in connect 4
On the other hand, the function to know if there is a tie in this game uses a function that runs through the entire matrix. If an empty space is found, it means that it is not yet a tie, so false
is returned.
In case of finishing going through the entire matrix and not finding an empty space, true
is returned.
Connect 4 artificial intelligence
As I said earlier, this game supports playing against the CPU. I have programmed a small artificial intelligence that chooses the best column based on the algorithm to try to win connect 4.
First it is verified if it is the turn of the CPU and if the game mode is player versus CPU. Then the best column is chosen and the value is placed on the board, (that is, the piece is placed).
After that, the state of the game is checked to see if there is a draw or a winner. If there is no winner or tie, the player is exchanged and it is the human’s turn.
The functions that allow you to choose the best column are shown below and are based on a series of rules:
What is done is to simulate a movement on the board (first cloning the original, so we do not directly modify it) and from the movement, check:
- If there is a column for the CPU to win
- If there is a column for the human to win, to take it
- The highest score, to know where to place the piece to have more chances of winning
Putting it all together
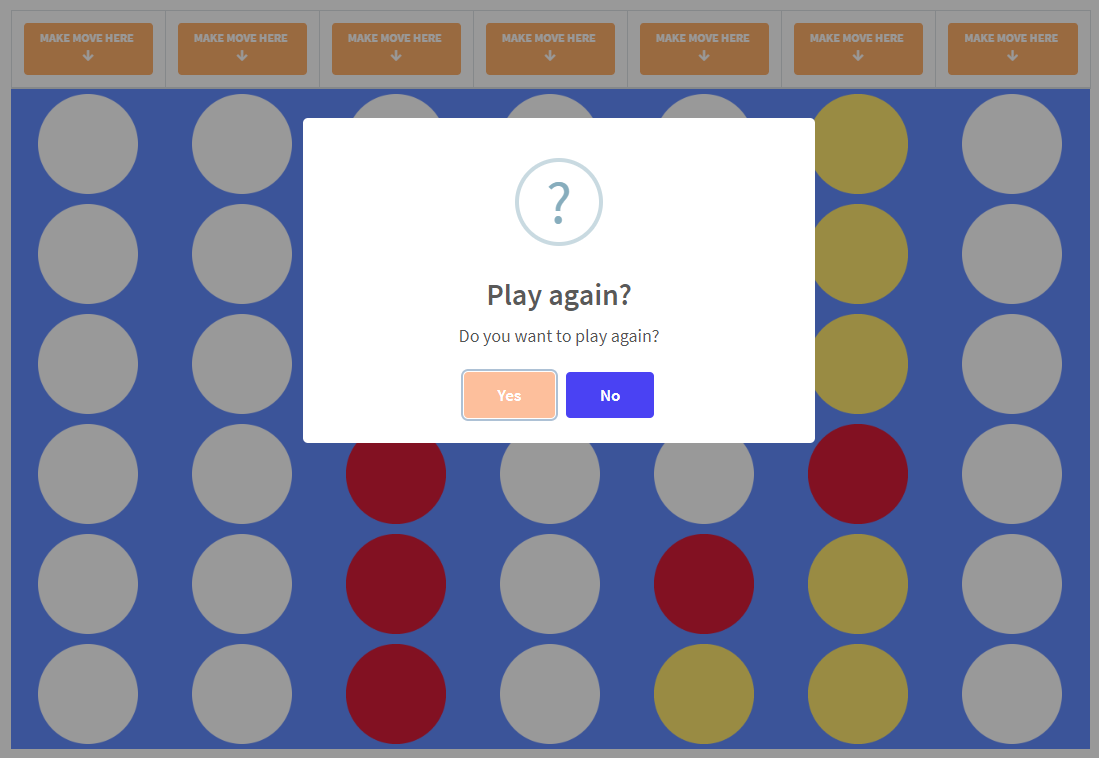
Finally, the complete JavaScript code is as seen below (I will leave the complete project at the end of the post).
I have not detailed some functions, it seems to me that they are very simple, for example, where the winner is shown or the game mode is asked, using Sweet Alert 2.
Downloads and source code
Like many of my projects, this software is free and open source. You can download the code from the GitHub repository.
Oh, and of course, you can see the demo right here.
Conclusion
I was quite excited to port this game to JavaScript with my favorite framework: Vue. Remember that previously I made this game in C language.
I’ve always liked how I made the CPU pick the best column and that, while it’s not perfect, can win sometimes.