When we migrate customers from MijoShop to OpenCart there is a little problem: the methods to hash passwords are different and thus when our customers try to login the passwords do not match.
In this post I will show you how to migrate and fix the problem.
Understanding the problem
OpenCart uses SHA1 and a random salt to store the password, but MijoShop uses different methods depending of the Joomla version. Sometimes it uses password_hash
methods, I mean, bcrypt.
So we only have to fix it on the login. First we check if the password (hashed) contains the string $P$
or $2
; the second one tell us that it was hashed with bcrypt.
In case it was hashed with SHA1 (OpenCart method), the hashed password will not contain any of these strings and then we leave OpenCart handle the login as usually.
But if the hash contains one of those strings, we check if it matches with two algorithms.
At the end, if the password matches in one of the three ways, we allow the user to login.
Code to fix migration from MijoShop to OpenCart
Note: remember that all of these modifications could be inside a ocmod/vqmod modification, here I’m just showing what to edit.
We must edit the file called customer.php, it’s located on system/library/cart/customer.php. In that file we will:
- Add a class called PasswordHash, obtained from Joomla source code
- Add a method called
joomla_check
to the Customer class, this will check the password hash and figure out which algoritmh was used. - Modify the login method to call the
joomla_check
Thanks to this forum thread I could figure out how to make OpenCart compatible with MijoShop. First we need the class that Joomla uses, and it’s as follow:
We must put it on an accesible place from customer.php (i put it inside the file):
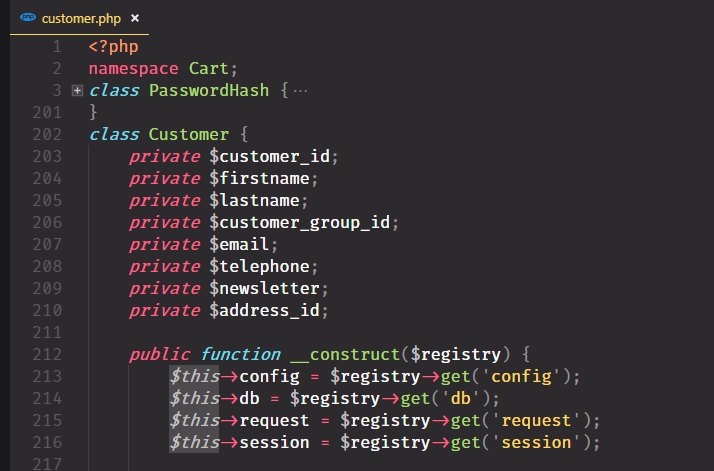
The class is minimized but it’s above Customer class. Then we add the private function joomla_check inside the Customer class:
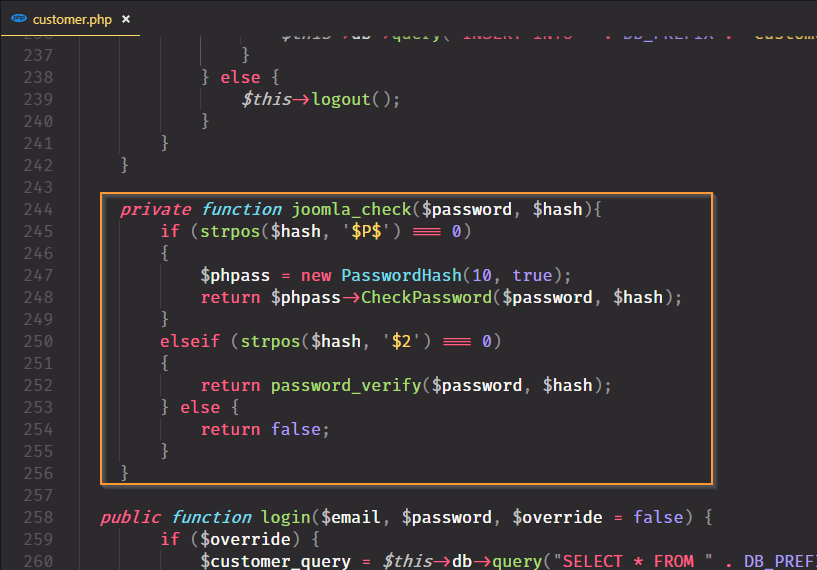
Finally we modify the login method adding the logic to check if the passwords belong to joomla:
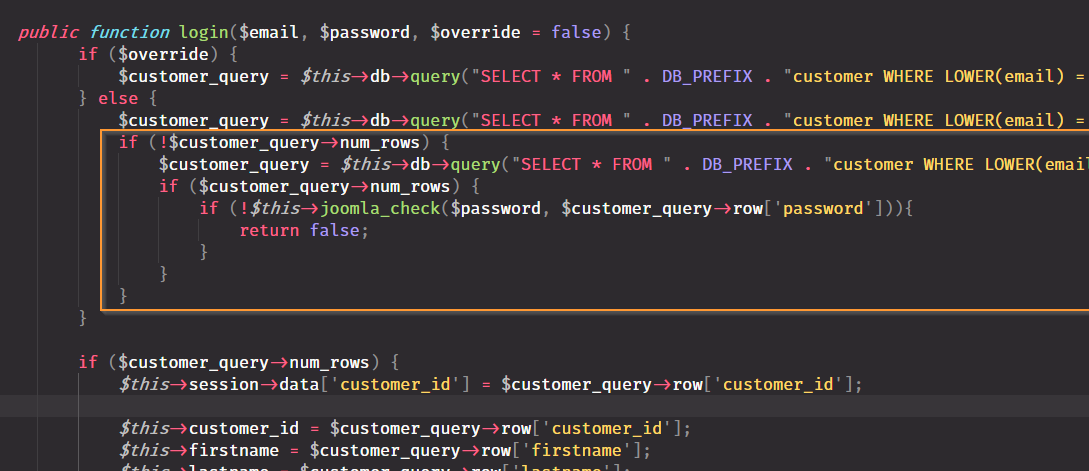
And we are ready. The final code looks like this:
Bonus: database consideration
I don’t know how are you migrating but remember that the hashes from joomla take more space. You can alter your SQL table, I’m using MySQL so:
In my case the prefix is oc_
because I chose it when I Install OpenCart.
Conclusion
In this way we can allow old customers from MijoShop to login on OpenCart, and the advantage is that new users will be able to login as well; so all the passwords (olds and news) are checked according to the hash.
Here you can read more posts about OpenCart.