Introduction
Today we will see how to convert a string in C to uppercase. We will also see how to convert a string to lowercase. That is, to convert something like “Hello World” to “hello world”. Or something like “Good night” to “GOOD NIGHT”.
For this we will use some functions that the standard library has: tolower
and toupper
.
ctype.h library in C
To use toupper and tolower in C we will need to include the library that defines it. We simply include it with #include
:
#include <ctype.h>
With this we can use toupper and tolower in C to convert strings.
Convert C string to uppercase
There is no direct way to convert a string to upper case; but there is a way to convert a char to a capital letter.
Remember that strings are only arrays of characters; so we can go through them and capitalize each of its elements. Then let’s see this program that defines a string and then converts it to uppercase to print it later:
First we define a string. We print it to show it in its original state. Then we do a for loop where we increase the index from 0 until we reach the termination character \0
For each element in the array we convert the capital letter using toupper
function that comes when we include ctype.h
The execution of the program is as follows:
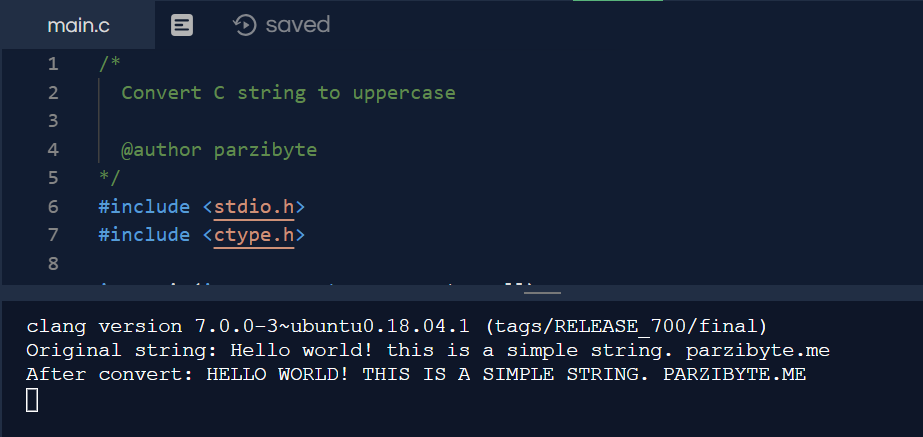
You can try it here.
Convert string to lowercase in C
This is almost the same as above, but now we use tolower
. The same code above, but to convert a string to lowercase, looks like this:
What we do is go through the string and turn each character into its lowercase representation.
Try it here.
Conclusion
This is how we can convert a string to uppercase or lowercase using the C language.